mirror of
https://gitlab.torproject.org/tpo/core/tor.git
synced 2024-11-10 13:13:44 +01:00
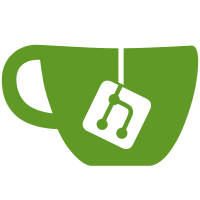
Otherwise I need to figure out what it does and how to make it do it every time I want to use it. It did that unixy thing where running it with no arguments printed nothing and returned.
67 lines
1.6 KiB
Perl
Executable File
67 lines
1.6 KiB
Perl
Executable File
#!/usr/bin/perl
|
|
|
|
use warnings;
|
|
use strict;
|
|
|
|
sub nChanges {
|
|
my ($branches, $fname) = @_;
|
|
local *F;
|
|
# requires perl 5.8. Avoids shell issues if we ever get a changes
|
|
# file named by the parents of Little Johnny Tables.
|
|
open F, "-|", "git", "log", "--pretty=format:%H", $branches, "--", $fname
|
|
or die "$!";
|
|
my @changes = <F>;
|
|
return scalar @changes
|
|
}
|
|
|
|
my $look_for_type = "merged";
|
|
|
|
if (! @ARGV) {
|
|
print <<EOF
|
|
Usage:
|
|
findMergedChanges.pl [--merged/--unmerged/--weird/--list] changes/*
|
|
|
|
A change is "merged" if it has ever been merged to release-0.2.2 and it has had
|
|
no subsequent changes in master.
|
|
|
|
A change is "unmerged" if it has never been merged to release-0.2.2 and it
|
|
has had changes in master.
|
|
|
|
A change is "weird" if it has been merged to release-0.2.2 and it *has* had
|
|
subsequent changes in master.
|
|
|
|
Suggested application:
|
|
findMergedChanges.pl --merged changes/* | xargs -n 1 git rm
|
|
|
|
EOF
|
|
}
|
|
|
|
while (@ARGV and $ARGV[0] =~ /^--/) {
|
|
my $flag = shift @ARGV;
|
|
if ($flag =~ /^--(weird|merged|unmerged|list)/) {
|
|
$look_for_type = $1;
|
|
} else {
|
|
die "Unrecognized flag $flag";
|
|
}
|
|
}
|
|
|
|
for my $changefile (@ARGV) {
|
|
my $n_merged = nChanges("origin/release-0.2.2", $changefile);
|
|
my $n_postmerged = nChanges("origin/release-0.2.2..origin/master", $changefile);
|
|
my $type;
|
|
|
|
if ($n_merged != 0 and $n_postmerged == 0) {
|
|
$type = "merged";
|
|
} elsif ($n_merged == 0 and $n_postmerged != 0) {
|
|
$type = "unmerged";
|
|
} else {
|
|
$type = "weird";
|
|
}
|
|
|
|
if ($type eq $look_for_type) {
|
|
print "$changefile\n";
|
|
} elsif ($look_for_type eq 'list') {
|
|
printf "% 8s: %s\n", $type, $changefile;
|
|
}
|
|
}
|