mirror of
https://gitlab.torproject.org/tpo/core/tor.git
synced 2024-11-10 21:23:58 +01:00
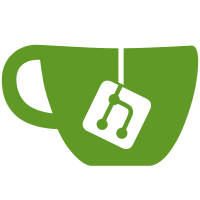
number of system-wide available file descriptors: /proc/sys/fs/file-max is bigger than 100k, set ulimit -n to 32k, if it's smaller than 20k set it to 1024. Big servers at the moment regularly use more than 10k FDs, so our old default of 8k no longer is sufficient. On the other hand we don't want lower end systems to run out of FDs on Tor's account. svn:r13625
185 lines
4.1 KiB
Bash
185 lines
4.1 KiB
Bash
#! /bin/bash
|
|
|
|
### BEGIN INIT INFO
|
|
# Provides: tor
|
|
# Required-Start: $local_fs $remote_fs $network $named $time
|
|
# Required-Stop: $local_fs $remote_fs $network $named $time
|
|
# Should-Start: $syslog
|
|
# Should-Stop: $syslog
|
|
# Default-Start: 2 3 4 5
|
|
# Default-Stop: 0 1 6
|
|
# Short-Description: Starts The Onion Router daemon processes
|
|
# Description: Start The Onion Router, a TCP overlay
|
|
# network client that provides anonymous
|
|
# transport.
|
|
### END INIT INFO
|
|
|
|
set -e
|
|
|
|
PATH=/usr/local/sbin:/usr/local/bin:/sbin:/bin:/usr/sbin:/usr/bin
|
|
DAEMON=/usr/sbin/tor
|
|
NAME=tor
|
|
DESC="tor daemon"
|
|
TORPIDDIR=/var/run/tor
|
|
TORPID=$TORPIDDIR/tor.pid
|
|
DEFAULTSFILE=/etc/default/$NAME
|
|
WAITFORDAEMON=60
|
|
ARGS=""
|
|
# Let's try to figure our some sane defaults:
|
|
if [ -r /proc/sys/fs/file-max ]; then
|
|
system_max=`cat /proc/sys/fs/file-max`
|
|
if [ "$system_max" -gt "100000" ] ; then
|
|
MAX_FILEDESCRIPTORS=32768
|
|
elif [ "$system_max" -gt "20000" ] ; then
|
|
MAX_FILEDESCRIPTORS=8192
|
|
else
|
|
MAX_FILEDESCRIPTORS=1024
|
|
fi
|
|
else
|
|
MAX_FILEDESCRIPTORS=8192
|
|
fi
|
|
|
|
NICE=""
|
|
|
|
test -x $DAEMON || exit 0
|
|
|
|
# Include tor defaults if available
|
|
if [ -f $DEFAULTSFILE ] ; then
|
|
. $DEFAULTSFILE
|
|
fi
|
|
|
|
wait_for_deaddaemon () {
|
|
pid=$1
|
|
sleep 1
|
|
if test -n "$pid"
|
|
then
|
|
if kill -0 $pid 2>/dev/null
|
|
then
|
|
echo -n "."
|
|
cnt=0
|
|
while kill -0 $pid 2>/dev/null
|
|
do
|
|
cnt=`expr $cnt + 1`
|
|
if [ $cnt -gt $WAITFORDAEMON ]
|
|
then
|
|
echo " FAILED."
|
|
return 1
|
|
fi
|
|
sleep 1
|
|
echo -n "."
|
|
done
|
|
fi
|
|
fi
|
|
return 0
|
|
}
|
|
|
|
|
|
check_torpiddir () {
|
|
if test ! -d $TORPIDDIR; then
|
|
echo "There is no $TORPIDDIR directory. Creating one for you."
|
|
mkdir -m 02700 "$TORPIDDIR"
|
|
chown debian-tor:debian-tor "$TORPIDDIR"
|
|
fi
|
|
|
|
if test ! -x $TORPIDDIR; then
|
|
echo "Cannot access $TORPIDDIR directory, are you root?" >&2
|
|
exit 1
|
|
fi
|
|
}
|
|
|
|
|
|
case "$1" in
|
|
start)
|
|
if [ "$RUN_DAEMON" != "yes" ]; then
|
|
echo "Not starting $DESC (Disabled in $DEFAULTSFILE)."
|
|
exit 0
|
|
fi
|
|
|
|
if [ -n "$MAX_FILEDESCRIPTORS" ]; then
|
|
echo -n "Raising maximum number of filedescriptors (ulimit -n) to $MAX_FILEDESCRIPTORS"
|
|
if ulimit -n "$MAX_FILEDESCRIPTORS" ; then
|
|
echo "."
|
|
else
|
|
echo ": FAILED."
|
|
fi
|
|
fi
|
|
|
|
check_torpiddir
|
|
|
|
echo "Starting $DESC: $NAME..."
|
|
if ! su -s /bin/sh -c "$DAEMON --verify-config" debian-tor > /dev/null; then
|
|
echo "ABORTED: Tor configuration invalid:" >&2
|
|
su -s /bin/sh -c "$DAEMON --verify-config" debian-tor >&2
|
|
exit 1
|
|
fi
|
|
|
|
start-stop-daemon --start --quiet --oknodo \
|
|
--chuid debian-tor:debian-tor \
|
|
--pidfile $TORPID \
|
|
$NICE \
|
|
--exec $DAEMON -- $ARGS
|
|
echo "done."
|
|
;;
|
|
stop)
|
|
echo -n "Stopping $DESC: "
|
|
pid=`cat $TORPID 2>/dev/null` || true
|
|
|
|
if test ! -f $TORPID -o -z "$pid"; then
|
|
echo "not running (there is no $TORPID)."
|
|
exit 0
|
|
fi
|
|
|
|
if start-stop-daemon --stop --signal INT --quiet --pidfile $TORPID --exec $DAEMON; then
|
|
wait_for_deaddaemon $pid
|
|
echo "$NAME."
|
|
elif kill -0 $pid 2>/dev/null
|
|
then
|
|
echo "FAILED (Is $pid not $NAME? Is $DAEMON a different binary now?)."
|
|
else
|
|
echo "FAILED ($DAEMON died: process $pid not running; or permission denied)."
|
|
fi
|
|
;;
|
|
reload|force-reload)
|
|
echo -n "Reloading $DESC configuration: "
|
|
pid=`cat $TORPID 2>/dev/null` || true
|
|
|
|
if test ! -f $TORPID -o -z "$pid"; then
|
|
echo "not running (there is no $TORPID)."
|
|
exit 0
|
|
fi
|
|
|
|
if ! su -s /bin/sh -c "$DAEMON --verify-config" debian-tor > /dev/null; then
|
|
echo "ABORTED: Tor configuration invalid:" >&2
|
|
su -s /bin/sh -c "$DAEMON --verify-config" debian-tor >&2
|
|
exit 1
|
|
fi
|
|
|
|
if start-stop-daemon --stop --signal 1 --quiet --pidfile $TORPID --exec $DAEMON
|
|
then
|
|
echo "$NAME."
|
|
elif kill -0 $pid 2>/dev/null
|
|
then
|
|
echo "FAILED (Is $pid not $NAME? Is $DAEMON a different binary now?)."
|
|
else
|
|
echo "FAILED ($DAEMON died: process $pid not running; or permission denied)."
|
|
fi
|
|
;;
|
|
restart)
|
|
if ! su -s /bin/sh -c "$DAEMON --verify-config" debian-tor > /dev/null; then
|
|
echo "Restarting Tor ABORTED: Tor configuration invalid:" >&2
|
|
su -s /bin/sh -c "$DAEMON --verify-config" debian-tor >&2
|
|
exit 1
|
|
fi
|
|
|
|
$0 stop
|
|
sleep 1
|
|
$0 start
|
|
;;
|
|
*)
|
|
echo "Usage: $0 {start|stop|restart|reload|force-reload}" >&2
|
|
exit 1
|
|
;;
|
|
esac
|
|
|
|
exit 0
|