mirror of
https://gitlab.torproject.org/tpo/core/tor.git
synced 2024-11-11 13:43:47 +01:00
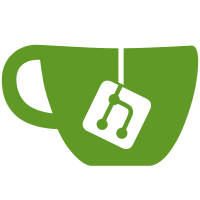
This commit makes tor add the DoS cell extension to the ESTABLISH_INTRO cell if the defense is enabled on the service side with a torrc option. Furthermore, the cell extension is only added if the introduction point supports it. The protover version HSIntro=5 is looked for. Signed-off-by: David Goulet <dgoulet@torproject.org>
436 lines
22 KiB
C
436 lines
22 KiB
C
/* cell_establish_intro.h -- generated by Trunnel v1.5.3.
|
|
* https://gitweb.torproject.org/trunnel.git
|
|
* You probably shouldn't edit this file.
|
|
*/
|
|
#ifndef TRUNNEL_CELL_ESTABLISH_INTRO_H
|
|
#define TRUNNEL_CELL_ESTABLISH_INTRO_H
|
|
|
|
#include <stdint.h>
|
|
#include "trunnel.h"
|
|
|
|
struct trn_cell_extension_st;
|
|
#define TRUNNEL_SHA3_256_LEN 32
|
|
#define TRUNNEL_CELL_EXTENSION_TYPE_DOS 1
|
|
#define TRUNNEL_DOS_PARAM_TYPE_INTRO2_RATE_PER_SEC 1
|
|
#define TRUNNEL_DOS_PARAM_TYPE_INTRO2_BURST_PER_SEC 2
|
|
#if !defined(TRUNNEL_OPAQUE) && !defined(TRUNNEL_OPAQUE_TRN_CELL_EXTENSION_DOS_PARAM)
|
|
struct trn_cell_extension_dos_param_st {
|
|
uint8_t type;
|
|
uint64_t value;
|
|
uint8_t trunnel_error_code_;
|
|
};
|
|
#endif
|
|
typedef struct trn_cell_extension_dos_param_st trn_cell_extension_dos_param_t;
|
|
#if !defined(TRUNNEL_OPAQUE) && !defined(TRUNNEL_OPAQUE_TRN_CELL_ESTABLISH_INTRO)
|
|
struct trn_cell_establish_intro_st {
|
|
const uint8_t *start_cell;
|
|
uint8_t auth_key_type;
|
|
uint16_t auth_key_len;
|
|
TRUNNEL_DYNARRAY_HEAD(, uint8_t) auth_key;
|
|
struct trn_cell_extension_st *extensions;
|
|
const uint8_t *end_mac_fields;
|
|
uint8_t handshake_mac[TRUNNEL_SHA3_256_LEN];
|
|
const uint8_t *end_sig_fields;
|
|
uint16_t sig_len;
|
|
TRUNNEL_DYNARRAY_HEAD(, uint8_t) sig;
|
|
uint8_t trunnel_error_code_;
|
|
};
|
|
#endif
|
|
typedef struct trn_cell_establish_intro_st trn_cell_establish_intro_t;
|
|
#if !defined(TRUNNEL_OPAQUE) && !defined(TRUNNEL_OPAQUE_TRN_CELL_EXTENSION_DOS)
|
|
struct trn_cell_extension_dos_st {
|
|
uint8_t n_params;
|
|
TRUNNEL_DYNARRAY_HEAD(, struct trn_cell_extension_dos_param_st *) params;
|
|
uint8_t trunnel_error_code_;
|
|
};
|
|
#endif
|
|
typedef struct trn_cell_extension_dos_st trn_cell_extension_dos_t;
|
|
#if !defined(TRUNNEL_OPAQUE) && !defined(TRUNNEL_OPAQUE_TRN_CELL_INTRO_ESTABLISHED)
|
|
struct trn_cell_intro_established_st {
|
|
struct trn_cell_extension_st *extensions;
|
|
uint8_t trunnel_error_code_;
|
|
};
|
|
#endif
|
|
typedef struct trn_cell_intro_established_st trn_cell_intro_established_t;
|
|
/** Return a newly allocated trn_cell_extension_dos_param with all
|
|
* elements set to zero.
|
|
*/
|
|
trn_cell_extension_dos_param_t *trn_cell_extension_dos_param_new(void);
|
|
/** Release all storage held by the trn_cell_extension_dos_param in
|
|
* 'victim'. (Do nothing if 'victim' is NULL.)
|
|
*/
|
|
void trn_cell_extension_dos_param_free(trn_cell_extension_dos_param_t *victim);
|
|
/** Try to parse a trn_cell_extension_dos_param from the buffer in
|
|
* 'input', using up to 'len_in' bytes from the input buffer. On
|
|
* success, return the number of bytes consumed and set *output to the
|
|
* newly allocated trn_cell_extension_dos_param_t. On failure, return
|
|
* -2 if the input appears truncated, and -1 if the input is otherwise
|
|
* invalid.
|
|
*/
|
|
ssize_t trn_cell_extension_dos_param_parse(trn_cell_extension_dos_param_t **output, const uint8_t *input, const size_t len_in);
|
|
/** Return the number of bytes we expect to need to encode the
|
|
* trn_cell_extension_dos_param in 'obj'. On failure, return a
|
|
* negative value. Note that this value may be an overestimate, and
|
|
* can even be an underestimate for certain unencodeable objects.
|
|
*/
|
|
ssize_t trn_cell_extension_dos_param_encoded_len(const trn_cell_extension_dos_param_t *obj);
|
|
/** Try to encode the trn_cell_extension_dos_param from 'input' into
|
|
* the buffer at 'output', using up to 'avail' bytes of the output
|
|
* buffer. On success, return the number of bytes used. On failure,
|
|
* return -2 if the buffer was not long enough, and -1 if the input
|
|
* was invalid.
|
|
*/
|
|
ssize_t trn_cell_extension_dos_param_encode(uint8_t *output, size_t avail, const trn_cell_extension_dos_param_t *input);
|
|
/** Check whether the internal state of the
|
|
* trn_cell_extension_dos_param in 'obj' is consistent. Return NULL if
|
|
* it is, and a short message if it is not.
|
|
*/
|
|
const char *trn_cell_extension_dos_param_check(const trn_cell_extension_dos_param_t *obj);
|
|
/** Clear any errors that were set on the object 'obj' by its setter
|
|
* functions. Return true iff errors were cleared.
|
|
*/
|
|
int trn_cell_extension_dos_param_clear_errors(trn_cell_extension_dos_param_t *obj);
|
|
/** Return the value of the type field of the
|
|
* trn_cell_extension_dos_param_t in 'inp'
|
|
*/
|
|
uint8_t trn_cell_extension_dos_param_get_type(const trn_cell_extension_dos_param_t *inp);
|
|
/** Set the value of the type field of the
|
|
* trn_cell_extension_dos_param_t in 'inp' to 'val'. Return 0 on
|
|
* success; return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_extension_dos_param_set_type(trn_cell_extension_dos_param_t *inp, uint8_t val);
|
|
/** Return the value of the value field of the
|
|
* trn_cell_extension_dos_param_t in 'inp'
|
|
*/
|
|
uint64_t trn_cell_extension_dos_param_get_value(const trn_cell_extension_dos_param_t *inp);
|
|
/** Set the value of the value field of the
|
|
* trn_cell_extension_dos_param_t in 'inp' to 'val'. Return 0 on
|
|
* success; return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_extension_dos_param_set_value(trn_cell_extension_dos_param_t *inp, uint64_t val);
|
|
/** Return a newly allocated trn_cell_establish_intro with all
|
|
* elements set to zero.
|
|
*/
|
|
trn_cell_establish_intro_t *trn_cell_establish_intro_new(void);
|
|
/** Release all storage held by the trn_cell_establish_intro in
|
|
* 'victim'. (Do nothing if 'victim' is NULL.)
|
|
*/
|
|
void trn_cell_establish_intro_free(trn_cell_establish_intro_t *victim);
|
|
/** Try to parse a trn_cell_establish_intro from the buffer in
|
|
* 'input', using up to 'len_in' bytes from the input buffer. On
|
|
* success, return the number of bytes consumed and set *output to the
|
|
* newly allocated trn_cell_establish_intro_t. On failure, return -2
|
|
* if the input appears truncated, and -1 if the input is otherwise
|
|
* invalid.
|
|
*/
|
|
ssize_t trn_cell_establish_intro_parse(trn_cell_establish_intro_t **output, const uint8_t *input, const size_t len_in);
|
|
/** Return the number of bytes we expect to need to encode the
|
|
* trn_cell_establish_intro in 'obj'. On failure, return a negative
|
|
* value. Note that this value may be an overestimate, and can even be
|
|
* an underestimate for certain unencodeable objects.
|
|
*/
|
|
ssize_t trn_cell_establish_intro_encoded_len(const trn_cell_establish_intro_t *obj);
|
|
/** Try to encode the trn_cell_establish_intro from 'input' into the
|
|
* buffer at 'output', using up to 'avail' bytes of the output buffer.
|
|
* On success, return the number of bytes used. On failure, return -2
|
|
* if the buffer was not long enough, and -1 if the input was invalid.
|
|
*/
|
|
ssize_t trn_cell_establish_intro_encode(uint8_t *output, size_t avail, const trn_cell_establish_intro_t *input);
|
|
/** Check whether the internal state of the trn_cell_establish_intro
|
|
* in 'obj' is consistent. Return NULL if it is, and a short message
|
|
* if it is not.
|
|
*/
|
|
const char *trn_cell_establish_intro_check(const trn_cell_establish_intro_t *obj);
|
|
/** Clear any errors that were set on the object 'obj' by its setter
|
|
* functions. Return true iff errors were cleared.
|
|
*/
|
|
int trn_cell_establish_intro_clear_errors(trn_cell_establish_intro_t *obj);
|
|
/** Return the position for start_cell when we parsed this object
|
|
*/
|
|
const uint8_t * trn_cell_establish_intro_get_start_cell(const trn_cell_establish_intro_t *inp);
|
|
/** Return the value of the auth_key_type field of the
|
|
* trn_cell_establish_intro_t in 'inp'
|
|
*/
|
|
uint8_t trn_cell_establish_intro_get_auth_key_type(const trn_cell_establish_intro_t *inp);
|
|
/** Set the value of the auth_key_type field of the
|
|
* trn_cell_establish_intro_t in 'inp' to 'val'. Return 0 on success;
|
|
* return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_establish_intro_set_auth_key_type(trn_cell_establish_intro_t *inp, uint8_t val);
|
|
/** Return the value of the auth_key_len field of the
|
|
* trn_cell_establish_intro_t in 'inp'
|
|
*/
|
|
uint16_t trn_cell_establish_intro_get_auth_key_len(const trn_cell_establish_intro_t *inp);
|
|
/** Set the value of the auth_key_len field of the
|
|
* trn_cell_establish_intro_t in 'inp' to 'val'. Return 0 on success;
|
|
* return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_establish_intro_set_auth_key_len(trn_cell_establish_intro_t *inp, uint16_t val);
|
|
/** Return the length of the dynamic array holding the auth_key field
|
|
* of the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
size_t trn_cell_establish_intro_getlen_auth_key(const trn_cell_establish_intro_t *inp);
|
|
/** Return the element at position 'idx' of the dynamic array field
|
|
* auth_key of the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
uint8_t trn_cell_establish_intro_get_auth_key(trn_cell_establish_intro_t *inp, size_t idx);
|
|
/** As trn_cell_establish_intro_get_auth_key, but take and return a
|
|
* const pointer
|
|
*/
|
|
uint8_t trn_cell_establish_intro_getconst_auth_key(const trn_cell_establish_intro_t *inp, size_t idx);
|
|
/** Change the element at position 'idx' of the dynamic array field
|
|
* auth_key of the trn_cell_establish_intro_t in 'inp', so that it
|
|
* will hold the value 'elt'.
|
|
*/
|
|
int trn_cell_establish_intro_set_auth_key(trn_cell_establish_intro_t *inp, size_t idx, uint8_t elt);
|
|
/** Append a new element 'elt' to the dynamic array field auth_key of
|
|
* the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
int trn_cell_establish_intro_add_auth_key(trn_cell_establish_intro_t *inp, uint8_t elt);
|
|
/** Return a pointer to the variable-length array field auth_key of
|
|
* 'inp'.
|
|
*/
|
|
uint8_t * trn_cell_establish_intro_getarray_auth_key(trn_cell_establish_intro_t *inp);
|
|
/** As trn_cell_establish_intro_get_auth_key, but take and return a
|
|
* const pointer
|
|
*/
|
|
const uint8_t * trn_cell_establish_intro_getconstarray_auth_key(const trn_cell_establish_intro_t *inp);
|
|
/** Change the length of the variable-length array field auth_key of
|
|
* 'inp' to 'newlen'.Fill extra elements with 0. Return 0 on success;
|
|
* return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_establish_intro_setlen_auth_key(trn_cell_establish_intro_t *inp, size_t newlen);
|
|
/** Return the value of the extensions field of the
|
|
* trn_cell_establish_intro_t in 'inp'
|
|
*/
|
|
struct trn_cell_extension_st * trn_cell_establish_intro_get_extensions(trn_cell_establish_intro_t *inp);
|
|
/** As trn_cell_establish_intro_get_extensions, but take and return a
|
|
* const pointer
|
|
*/
|
|
const struct trn_cell_extension_st * trn_cell_establish_intro_getconst_extensions(const trn_cell_establish_intro_t *inp);
|
|
/** Set the value of the extensions field of the
|
|
* trn_cell_establish_intro_t in 'inp' to 'val'. Free the old value if
|
|
* any. Steals the referenceto 'val'.Return 0 on success; return -1
|
|
* and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_establish_intro_set_extensions(trn_cell_establish_intro_t *inp, struct trn_cell_extension_st *val);
|
|
/** As trn_cell_establish_intro_set_extensions, but does not free the
|
|
* previous value.
|
|
*/
|
|
int trn_cell_establish_intro_set0_extensions(trn_cell_establish_intro_t *inp, struct trn_cell_extension_st *val);
|
|
/** Return the position for end_mac_fields when we parsed this object
|
|
*/
|
|
const uint8_t * trn_cell_establish_intro_get_end_mac_fields(const trn_cell_establish_intro_t *inp);
|
|
/** Return the (constant) length of the array holding the
|
|
* handshake_mac field of the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
size_t trn_cell_establish_intro_getlen_handshake_mac(const trn_cell_establish_intro_t *inp);
|
|
/** Return the element at position 'idx' of the fixed array field
|
|
* handshake_mac of the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
uint8_t trn_cell_establish_intro_get_handshake_mac(trn_cell_establish_intro_t *inp, size_t idx);
|
|
/** As trn_cell_establish_intro_get_handshake_mac, but take and return
|
|
* a const pointer
|
|
*/
|
|
uint8_t trn_cell_establish_intro_getconst_handshake_mac(const trn_cell_establish_intro_t *inp, size_t idx);
|
|
/** Change the element at position 'idx' of the fixed array field
|
|
* handshake_mac of the trn_cell_establish_intro_t in 'inp', so that
|
|
* it will hold the value 'elt'.
|
|
*/
|
|
int trn_cell_establish_intro_set_handshake_mac(trn_cell_establish_intro_t *inp, size_t idx, uint8_t elt);
|
|
/** Return a pointer to the TRUNNEL_SHA3_256_LEN-element array field
|
|
* handshake_mac of 'inp'.
|
|
*/
|
|
uint8_t * trn_cell_establish_intro_getarray_handshake_mac(trn_cell_establish_intro_t *inp);
|
|
/** As trn_cell_establish_intro_get_handshake_mac, but take and return
|
|
* a const pointer
|
|
*/
|
|
const uint8_t * trn_cell_establish_intro_getconstarray_handshake_mac(const trn_cell_establish_intro_t *inp);
|
|
/** Return the position for end_sig_fields when we parsed this object
|
|
*/
|
|
const uint8_t * trn_cell_establish_intro_get_end_sig_fields(const trn_cell_establish_intro_t *inp);
|
|
/** Return the value of the sig_len field of the
|
|
* trn_cell_establish_intro_t in 'inp'
|
|
*/
|
|
uint16_t trn_cell_establish_intro_get_sig_len(const trn_cell_establish_intro_t *inp);
|
|
/** Set the value of the sig_len field of the
|
|
* trn_cell_establish_intro_t in 'inp' to 'val'. Return 0 on success;
|
|
* return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_establish_intro_set_sig_len(trn_cell_establish_intro_t *inp, uint16_t val);
|
|
/** Return the length of the dynamic array holding the sig field of
|
|
* the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
size_t trn_cell_establish_intro_getlen_sig(const trn_cell_establish_intro_t *inp);
|
|
/** Return the element at position 'idx' of the dynamic array field
|
|
* sig of the trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
uint8_t trn_cell_establish_intro_get_sig(trn_cell_establish_intro_t *inp, size_t idx);
|
|
/** As trn_cell_establish_intro_get_sig, but take and return a const
|
|
* pointer
|
|
*/
|
|
uint8_t trn_cell_establish_intro_getconst_sig(const trn_cell_establish_intro_t *inp, size_t idx);
|
|
/** Change the element at position 'idx' of the dynamic array field
|
|
* sig of the trn_cell_establish_intro_t in 'inp', so that it will
|
|
* hold the value 'elt'.
|
|
*/
|
|
int trn_cell_establish_intro_set_sig(trn_cell_establish_intro_t *inp, size_t idx, uint8_t elt);
|
|
/** Append a new element 'elt' to the dynamic array field sig of the
|
|
* trn_cell_establish_intro_t in 'inp'.
|
|
*/
|
|
int trn_cell_establish_intro_add_sig(trn_cell_establish_intro_t *inp, uint8_t elt);
|
|
/** Return a pointer to the variable-length array field sig of 'inp'.
|
|
*/
|
|
uint8_t * trn_cell_establish_intro_getarray_sig(trn_cell_establish_intro_t *inp);
|
|
/** As trn_cell_establish_intro_get_sig, but take and return a const
|
|
* pointer
|
|
*/
|
|
const uint8_t * trn_cell_establish_intro_getconstarray_sig(const trn_cell_establish_intro_t *inp);
|
|
/** Change the length of the variable-length array field sig of 'inp'
|
|
* to 'newlen'.Fill extra elements with 0. Return 0 on success; return
|
|
* -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_establish_intro_setlen_sig(trn_cell_establish_intro_t *inp, size_t newlen);
|
|
/** Return a newly allocated trn_cell_extension_dos with all elements
|
|
* set to zero.
|
|
*/
|
|
trn_cell_extension_dos_t *trn_cell_extension_dos_new(void);
|
|
/** Release all storage held by the trn_cell_extension_dos in
|
|
* 'victim'. (Do nothing if 'victim' is NULL.)
|
|
*/
|
|
void trn_cell_extension_dos_free(trn_cell_extension_dos_t *victim);
|
|
/** Try to parse a trn_cell_extension_dos from the buffer in 'input',
|
|
* using up to 'len_in' bytes from the input buffer. On success,
|
|
* return the number of bytes consumed and set *output to the newly
|
|
* allocated trn_cell_extension_dos_t. On failure, return -2 if the
|
|
* input appears truncated, and -1 if the input is otherwise invalid.
|
|
*/
|
|
ssize_t trn_cell_extension_dos_parse(trn_cell_extension_dos_t **output, const uint8_t *input, const size_t len_in);
|
|
/** Return the number of bytes we expect to need to encode the
|
|
* trn_cell_extension_dos in 'obj'. On failure, return a negative
|
|
* value. Note that this value may be an overestimate, and can even be
|
|
* an underestimate for certain unencodeable objects.
|
|
*/
|
|
ssize_t trn_cell_extension_dos_encoded_len(const trn_cell_extension_dos_t *obj);
|
|
/** Try to encode the trn_cell_extension_dos from 'input' into the
|
|
* buffer at 'output', using up to 'avail' bytes of the output buffer.
|
|
* On success, return the number of bytes used. On failure, return -2
|
|
* if the buffer was not long enough, and -1 if the input was invalid.
|
|
*/
|
|
ssize_t trn_cell_extension_dos_encode(uint8_t *output, size_t avail, const trn_cell_extension_dos_t *input);
|
|
/** Check whether the internal state of the trn_cell_extension_dos in
|
|
* 'obj' is consistent. Return NULL if it is, and a short message if
|
|
* it is not.
|
|
*/
|
|
const char *trn_cell_extension_dos_check(const trn_cell_extension_dos_t *obj);
|
|
/** Clear any errors that were set on the object 'obj' by its setter
|
|
* functions. Return true iff errors were cleared.
|
|
*/
|
|
int trn_cell_extension_dos_clear_errors(trn_cell_extension_dos_t *obj);
|
|
/** Return the value of the n_params field of the
|
|
* trn_cell_extension_dos_t in 'inp'
|
|
*/
|
|
uint8_t trn_cell_extension_dos_get_n_params(const trn_cell_extension_dos_t *inp);
|
|
/** Set the value of the n_params field of the
|
|
* trn_cell_extension_dos_t in 'inp' to 'val'. Return 0 on success;
|
|
* return -1 and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_extension_dos_set_n_params(trn_cell_extension_dos_t *inp, uint8_t val);
|
|
/** Return the length of the dynamic array holding the params field of
|
|
* the trn_cell_extension_dos_t in 'inp'.
|
|
*/
|
|
size_t trn_cell_extension_dos_getlen_params(const trn_cell_extension_dos_t *inp);
|
|
/** Return the element at position 'idx' of the dynamic array field
|
|
* params of the trn_cell_extension_dos_t in 'inp'.
|
|
*/
|
|
struct trn_cell_extension_dos_param_st * trn_cell_extension_dos_get_params(trn_cell_extension_dos_t *inp, size_t idx);
|
|
/** As trn_cell_extension_dos_get_params, but take and return a const
|
|
* pointer
|
|
*/
|
|
const struct trn_cell_extension_dos_param_st * trn_cell_extension_dos_getconst_params(const trn_cell_extension_dos_t *inp, size_t idx);
|
|
/** Change the element at position 'idx' of the dynamic array field
|
|
* params of the trn_cell_extension_dos_t in 'inp', so that it will
|
|
* hold the value 'elt'. Free the previous value, if any.
|
|
*/
|
|
int trn_cell_extension_dos_set_params(trn_cell_extension_dos_t *inp, size_t idx, struct trn_cell_extension_dos_param_st * elt);
|
|
/** As trn_cell_extension_dos_set_params, but does not free the
|
|
* previous value.
|
|
*/
|
|
int trn_cell_extension_dos_set0_params(trn_cell_extension_dos_t *inp, size_t idx, struct trn_cell_extension_dos_param_st * elt);
|
|
/** Append a new element 'elt' to the dynamic array field params of
|
|
* the trn_cell_extension_dos_t in 'inp'.
|
|
*/
|
|
int trn_cell_extension_dos_add_params(trn_cell_extension_dos_t *inp, struct trn_cell_extension_dos_param_st * elt);
|
|
/** Return a pointer to the variable-length array field params of
|
|
* 'inp'.
|
|
*/
|
|
struct trn_cell_extension_dos_param_st * * trn_cell_extension_dos_getarray_params(trn_cell_extension_dos_t *inp);
|
|
/** As trn_cell_extension_dos_get_params, but take and return a const
|
|
* pointer
|
|
*/
|
|
const struct trn_cell_extension_dos_param_st * const * trn_cell_extension_dos_getconstarray_params(const trn_cell_extension_dos_t *inp);
|
|
/** Change the length of the variable-length array field params of
|
|
* 'inp' to 'newlen'.Fill extra elements with NULL; free removed
|
|
* elements. Return 0 on success; return -1 and set the error code on
|
|
* 'inp' on failure.
|
|
*/
|
|
int trn_cell_extension_dos_setlen_params(trn_cell_extension_dos_t *inp, size_t newlen);
|
|
/** Return a newly allocated trn_cell_intro_established with all
|
|
* elements set to zero.
|
|
*/
|
|
trn_cell_intro_established_t *trn_cell_intro_established_new(void);
|
|
/** Release all storage held by the trn_cell_intro_established in
|
|
* 'victim'. (Do nothing if 'victim' is NULL.)
|
|
*/
|
|
void trn_cell_intro_established_free(trn_cell_intro_established_t *victim);
|
|
/** Try to parse a trn_cell_intro_established from the buffer in
|
|
* 'input', using up to 'len_in' bytes from the input buffer. On
|
|
* success, return the number of bytes consumed and set *output to the
|
|
* newly allocated trn_cell_intro_established_t. On failure, return -2
|
|
* if the input appears truncated, and -1 if the input is otherwise
|
|
* invalid.
|
|
*/
|
|
ssize_t trn_cell_intro_established_parse(trn_cell_intro_established_t **output, const uint8_t *input, const size_t len_in);
|
|
/** Return the number of bytes we expect to need to encode the
|
|
* trn_cell_intro_established in 'obj'. On failure, return a negative
|
|
* value. Note that this value may be an overestimate, and can even be
|
|
* an underestimate for certain unencodeable objects.
|
|
*/
|
|
ssize_t trn_cell_intro_established_encoded_len(const trn_cell_intro_established_t *obj);
|
|
/** Try to encode the trn_cell_intro_established from 'input' into the
|
|
* buffer at 'output', using up to 'avail' bytes of the output buffer.
|
|
* On success, return the number of bytes used. On failure, return -2
|
|
* if the buffer was not long enough, and -1 if the input was invalid.
|
|
*/
|
|
ssize_t trn_cell_intro_established_encode(uint8_t *output, size_t avail, const trn_cell_intro_established_t *input);
|
|
/** Check whether the internal state of the trn_cell_intro_established
|
|
* in 'obj' is consistent. Return NULL if it is, and a short message
|
|
* if it is not.
|
|
*/
|
|
const char *trn_cell_intro_established_check(const trn_cell_intro_established_t *obj);
|
|
/** Clear any errors that were set on the object 'obj' by its setter
|
|
* functions. Return true iff errors were cleared.
|
|
*/
|
|
int trn_cell_intro_established_clear_errors(trn_cell_intro_established_t *obj);
|
|
/** Return the value of the extensions field of the
|
|
* trn_cell_intro_established_t in 'inp'
|
|
*/
|
|
struct trn_cell_extension_st * trn_cell_intro_established_get_extensions(trn_cell_intro_established_t *inp);
|
|
/** As trn_cell_intro_established_get_extensions, but take and return
|
|
* a const pointer
|
|
*/
|
|
const struct trn_cell_extension_st * trn_cell_intro_established_getconst_extensions(const trn_cell_intro_established_t *inp);
|
|
/** Set the value of the extensions field of the
|
|
* trn_cell_intro_established_t in 'inp' to 'val'. Free the old value
|
|
* if any. Steals the referenceto 'val'.Return 0 on success; return -1
|
|
* and set the error code on 'inp' on failure.
|
|
*/
|
|
int trn_cell_intro_established_set_extensions(trn_cell_intro_established_t *inp, struct trn_cell_extension_st *val);
|
|
/** As trn_cell_intro_established_set_extensions, but does not free
|
|
* the previous value.
|
|
*/
|
|
int trn_cell_intro_established_set0_extensions(trn_cell_intro_established_t *inp, struct trn_cell_extension_st *val);
|
|
|
|
|
|
#endif
|