mirror of
https://gitlab.torproject.org/tpo/core/tor.git
synced 2024-11-11 13:43:47 +01:00
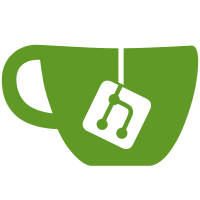
Previously, we only used the strong OS entropy source as part of seeding OpenSSL's RNG. But with curve25519, we'll have occasion to want to generate some keys using extremely-good entopy, as well as the means to do so. So let's! This patch refactors the OS-entropy wrapper into its own crypto_strongest_rand() function, and makes our new curve25519_secret_key_generate function try it as appropriate.
98 lines
3.2 KiB
C
98 lines
3.2 KiB
C
/* Copyright (c) 2012, The Tor Project, Inc. */
|
|
/* See LICENSE for licensing information */
|
|
|
|
/* Wrapper code for a curve25519 implementation. */
|
|
|
|
#define CRYPTO_CURVE25519_PRIVATE
|
|
#include "orconfig.h"
|
|
#include "crypto.h"
|
|
#include "crypto_curve25519.h"
|
|
#include "util.h"
|
|
|
|
/* ==============================
|
|
Part 1: wrap a suitable curve25519 implementation as curve25519_impl
|
|
============================== */
|
|
|
|
#ifdef USE_CURVE25519_DONNA
|
|
int curve25519_donna(uint8_t *mypublic,
|
|
const uint8_t *secret, const uint8_t *basepoint);
|
|
#endif
|
|
#ifdef USE_CURVE25519_NACL
|
|
#include <crypto_scalarmult_curve25519.h>
|
|
#endif
|
|
|
|
int
|
|
curve25519_impl(uint8_t *output, const uint8_t *secret,
|
|
const uint8_t *basepoint)
|
|
{
|
|
#ifdef USE_CURVE25519_DONNA
|
|
return curve25519_donna(output, secret, basepoint);
|
|
#elif defined(USE_CURVE25519_NACL)
|
|
return crypto_scalarmult_curve25519(output, secret, basepoint);
|
|
#else
|
|
#error "No implementation of curve25519 is available."
|
|
#endif
|
|
}
|
|
|
|
/* ==============================
|
|
Part 2: Wrap curve25519_impl with some convenience types and functions.
|
|
============================== */
|
|
|
|
/**
|
|
* Return true iff a curve25519_public_key_t seems valid. (It's not necessary
|
|
* to see if the point is on the curve, since the twist is also secure, but we
|
|
* do need to make sure that it isn't the point at infinity.) */
|
|
int
|
|
curve25519_public_key_is_ok(const curve25519_public_key_t *key)
|
|
{
|
|
static const uint8_t zero[] =
|
|
"\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"
|
|
"\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0";
|
|
|
|
return tor_memneq(key->public_key, zero, CURVE25519_PUBKEY_LEN);
|
|
}
|
|
|
|
/** Generate a new keypair and return the secret key. If <b>extra_strong</b>
|
|
* is true, this key is possibly going to get used more than once, so
|
|
* use a better-than-usual RNG. */
|
|
void
|
|
curve25519_secret_key_generate(curve25519_secret_key_t *key_out,
|
|
int extra_strong)
|
|
{
|
|
uint8_t k_tmp[CURVE25519_SECKEY_LEN];
|
|
|
|
crypto_rand((char*)key_out->secret_key, CURVE25519_SECKEY_LEN);
|
|
if (extra_strong && !crypto_strongest_rand(k_tmp, CURVE25519_SECKEY_LEN)) {
|
|
/* If they asked for extra-strong entropy and we have some, use it as an
|
|
* HMAC key to improve not-so-good entopy rather than using it directly,
|
|
* just in case the extra-strong entropy is less amazing than we hoped. */
|
|
crypto_hmac_sha256((char *)key_out->secret_key,
|
|
(const char *)k_tmp, sizeof(k_tmp),
|
|
(const char *)key_out->secret_key, CURVE25519_SECKEY_LEN);
|
|
}
|
|
memwipe(k_tmp, 0, sizeof(k_tmp));
|
|
key_out->secret_key[0] &= 248;
|
|
key_out->secret_key[31] &= 127;
|
|
key_out->secret_key[31] |= 64;
|
|
}
|
|
|
|
void
|
|
curve25519_public_key_generate(curve25519_public_key_t *key_out,
|
|
const curve25519_secret_key_t *seckey)
|
|
{
|
|
static const uint8_t basepoint[32] = {9};
|
|
|
|
curve25519_impl(key_out->public_key, seckey->secret_key, basepoint);
|
|
}
|
|
|
|
/** Perform the curve25519 ECDH handshake with <b>skey</b> and <b>pkey</b>,
|
|
* writing CURVE25519_OUTPUT_LEN bytes of output into <b>output</b>. */
|
|
void
|
|
curve25519_handshake(uint8_t *output,
|
|
const curve25519_secret_key_t *skey,
|
|
const curve25519_public_key_t *pkey)
|
|
{
|
|
curve25519_impl(output, skey->secret_key, pkey->public_key);
|
|
}
|
|
|